4.4 Iteration Statements
Loops allow a single statement or a statement block to be executed repeatedly (i.e., iterated). A boolean condition (called the loop condition) is commonly used to determine when to terminate the loop. The statements executed in the loop constitute the loop body.
Java provides four language constructs for loop construction:
- The while statement
- The do-while statement
- The basic for statement
- The enhanced for statement
These loops differ in the order in which they execute the loop body and test the loop condition. The while loop and the basic for loop test the loop condition before executing the loop body, whereas the do-while loop tests the loop condition after execution of the loop body.
The enhanced for loop (also called the for-each loop) simplifies iterating over arrays and collections. We will use the notations for(;;) and for(:) to designate the basic for loop and the enhanced for loop, respectively.
4.5 The while Statement
The syntax of the while loop is
while (
loop_condition
)
loop_body
The loop condition is evaluated before executing the loop body. The while statement executes the loop body as long as the loop condition is true. When the loop condition becomes false, the loop is terminated and execution continues with any statement immediately following the loop. If the loop condition is false to begin with, the loop body is not executed at all. In other words, a while loop can execute zero or more times. The loop condition must evaluate to a boolean or a Boolean value. In the latter case, the reference value is unboxed to a boolean value. The flow of control in a while statement is shown in Figure 4.6.
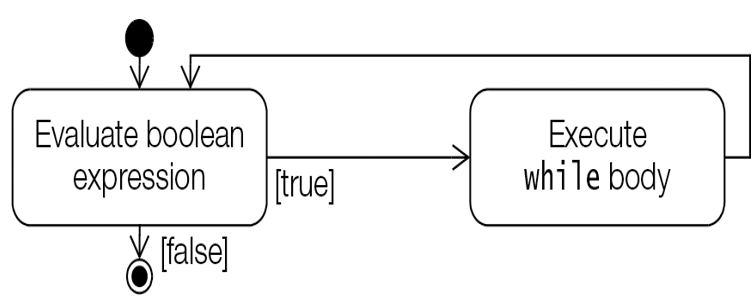
Figure 4.6 Activity Diagram for the while Statement
The while statement is normally used when the number of iterations is not known.
while (noSignOfLife())
keepLooking();
Since the loop body can be any valid statement, inadvertently terminating each line with the empty statement (;) can give unintended results. Always using a block statement as the loop body helps to avoid such problems.
while (noSignOfLife()); // Empty statement as loop body!
keepLooking(); // Statement not in the loop body.