4.1 Selection Statements
Java provides selection statements that allow the program to choose between alternative actions during execution. The choice is based on criteria specified in the selection statement. These selection statements are
- The simple if statement
- The if-else statement
- The switch statement and the switch expression
The Simple if Statement
The simple if statement has the following syntax:
if (
condition
)
statement
It is used to decide whether an action is to be performed or not, based on a condition. The action to be performed is specified by statement, which can be a single statement or a code block. The condition must evaluate to a boolean or Boolean value. In the latter case, the Boolean value is unboxed to the corresponding boolean value.
The semantics of the simple if statement are straightforward. The condition is evaluated first. If its value is true, statement (called the if block) is executed and then execution continues with the rest of the program. If the value is false, the if block is skipped and execution continues with the rest of the program. The semantics are illustrated by the activity diagram in Figure 4.1a.
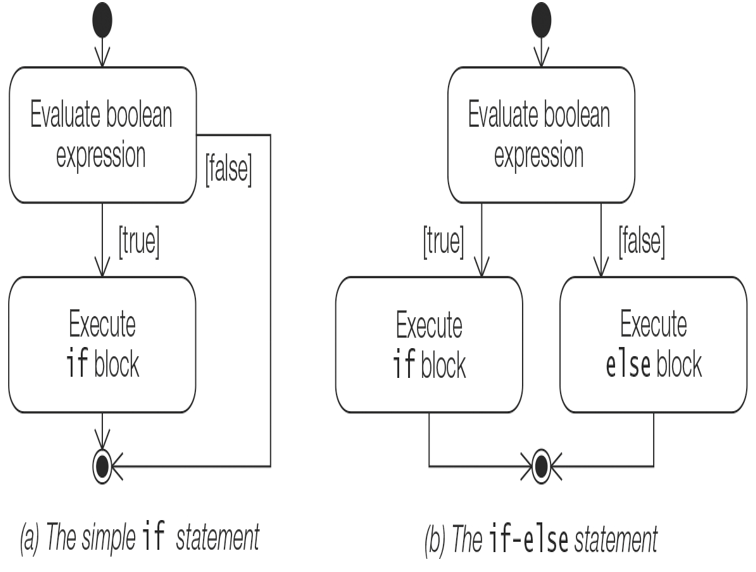
Figure 4.1 Activity Diagram for if Statements
In the following examples of the if statement, it is assumed that the variables and the methods have been appropriately defined:
if (emergency) // emergency is a boolean variable
operate();
if (temperature > critical)
soundAlarm();
if (isLeapYear() && endOfCentury())
celebrate();
if (catIsAway()) { // Block
getFishingRod();
goFishing();
}
Note that statement can be a block, and the block notation is necessary if more than one statement is to be executed when the condition is true.
Since the condition evaluates to a boolean value, it avoids a common programming error: using an expression of the form (a=b) as the condition, where inadvertently an assignment operator is used instead of a relational operator. The compiler will flag this as an error, unless both a and b are boolean.
Note that the if block can be any valid statement. In particular, it can be the empty statement (;) or the empty block ({}). A common programming error is inadvertent use of the empty statement.
if (emergency); // Empty if block
operate(); // Executed regardless of whether it was an emergency